TCP Port Scanner in Golang: A Revolutionary Guide
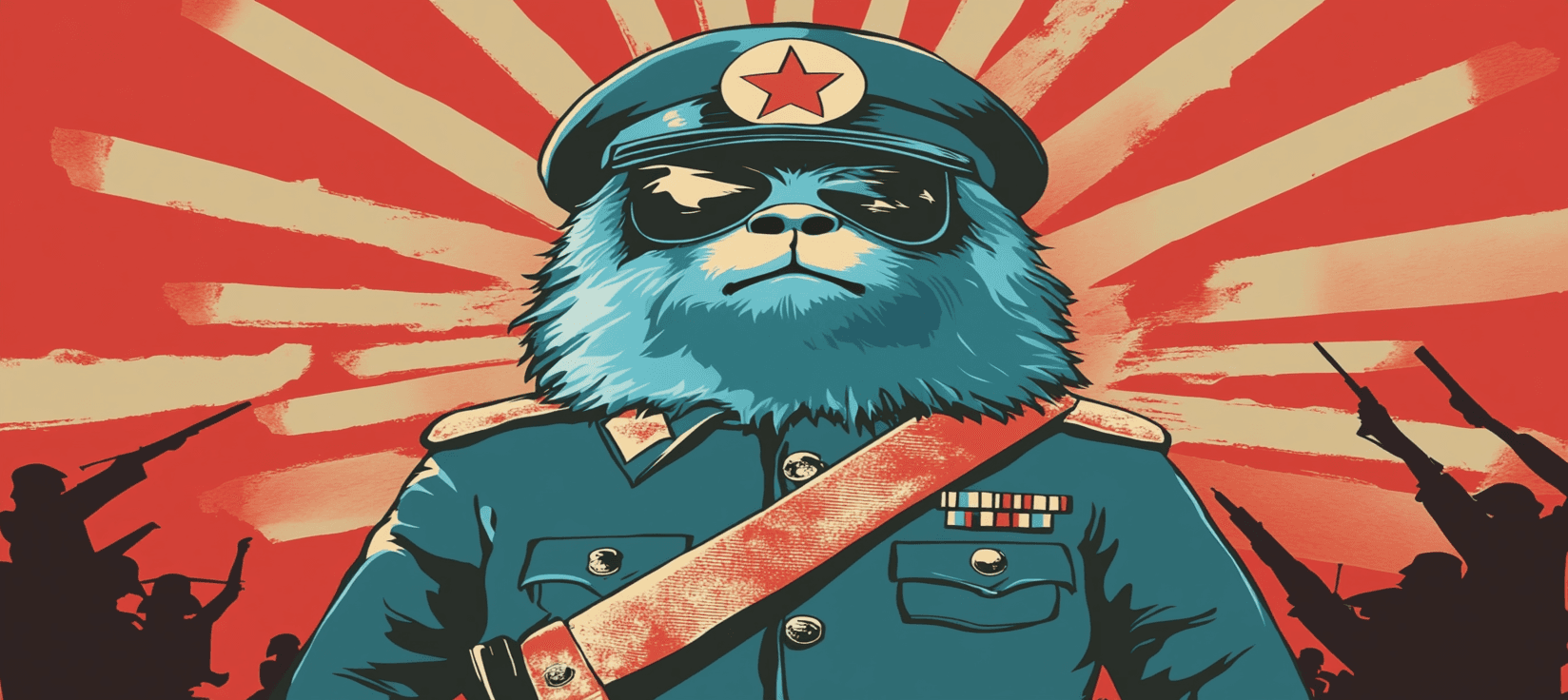
Imagine the internet as a bustling city, with every service, website, and app represented as buildings with doors. Each door is a port, and as software architects, we need the ability to knock on these doors to figure out which ones are open, closed, or outright ignoring us. Enter the mighty Port Scanner.
In today’s revolution-packed post, we’re going to dive into the nuts and bolts of building a TCP port scanner in Go, complete with unit tests and benchmarks. But before we storm the gates, let’s talk about the TCP handshake, the cornerstone of modern networking.
TCP Handshake: The Internet’s Secret Handshake
The TCP three-way handshake is like a formal introduction between two computers:
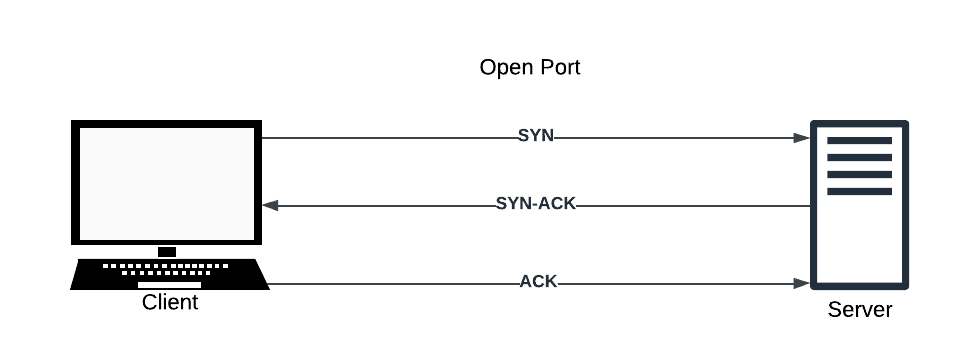
- SYN (Synchronize): The client says, "Hello, are you there?"
- SYN-ACK (Synchronize-Acknowledge): The server replies, "Yes, I’m here. Are you ready?"
- ACK (Acknowledge): The client confirms, "I’m ready. Let’s talk!"
If this handshake completes successfully, the port is open. If it doesn’t? The port could be closed, filtered, or the server might just not like your style.
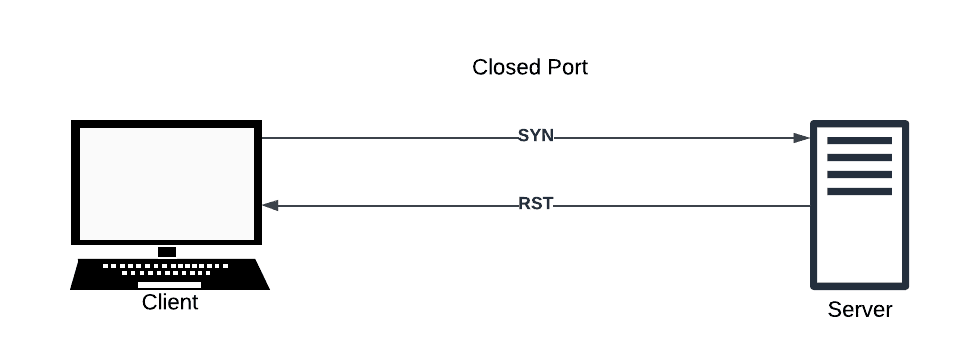
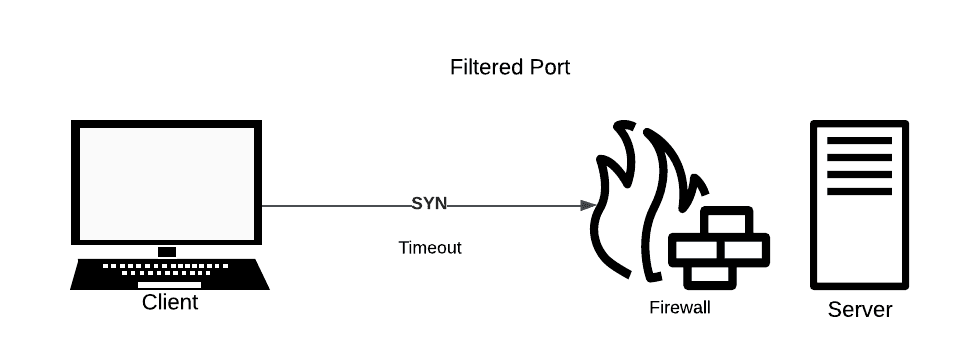
Building a Port Scanner in Go
Our port scanner in Go leverages the power of goroutines for concurrency and channels for efficient communication. Here’s what it does:
- Scans a range of ports on a given host.
- Uses TCP to determine if a port is open, closed, or times out.
- Supports configurable concurrency for speed.
- Includes throttling to avoid triggering alarms.
Scanner Core Functionality
Here’s a snippet of the scanner’s core functionality:
func (ps *PortScanner) Scan() error {
if err := ps.Validate(); err != nil {
return err
}
portCh := make(chan int, ps.Concurrency)
var wg sync.WaitGroup
for i := 0; i < ps.Concurrency; i++ {
wg.Add(1)
go func() {
defer wg.Done()
for port := range portCh {
status, err := ps.CheckPort(ps.Network, ps.Host, port)
ps.Results = append(ps.Results, PortStatus{
Port: port,
Status: status,
Error: func() string { if err != nil { return err.Error() } else { return "" } }(),
})
}
}()
}
for port := ps.StartPort; port <= ps.EndPort; port++ {
portCh <- port
}
close(portCh)
wg.Wait()
return nil
}
Testing the Scanner
No revolutionary project is complete without robust unit tests! We wrote tests for everything:
- Validation Logic: Ensures invalid configurations are caught early.
- Concurrency: Confirms no port is scanned twice.
- Result Consistency: Makes sure all ports are accounted for, errors or not.
Throttling Test
Here’s how we tested throttling to make sure our scanner doesn’t set off network alarms:
func TestPortScannerThrottle(t *testing.T) {
mockCheckPort := func(network, host string, port int) (string, error) {
return CLOSED, nil
}
scanner := PortScanner{
Host: "127.0.0.1",
StartPort: 1,
EndPort: 5,
Concurrency: 1,
Throttle: true,
CheckPort: mockCheckPort,
}
start := time.Now()
err := scanner.Scan()
if err != nil {
t.Fatalf("Scan() returned error: %v", err)
}
elapsed := time.Since(start)
if elapsed < 250*time.Millisecond {
t.Errorf("expected throttling to add delay; took %s", elapsed)
}
}
Benchmarking: The Race for Efficiency
To see how the scanner performs under different concurrency levels, we wrote this benchmark:
func BenchmarkPortScanner(b *testing.B) {
mockCheckPort := func(network, host string, port int) (string, error) {
return CLOSED, nil
}
for i := 1; i <= 10; i++ {
b.Run(fmt.Sprintf("Concurrency%d", i), func(b *testing.B) {
scanner := PortScanner{
Host: "127.0.0.1",
StartPort: 1,
EndPort: 100,
Concurrency: i,
Throttle: false,
CheckPort: mockCheckPort,
}
for n := 0; n < b.N; n++ {
_ = scanner.Scan()
}
})
}
}
Lessons Learned
- TCP Is Your Friend (and Foe): The handshake is simple, but firewalls and timeouts complicate things.
- Concurrency Is Powerful: Go’s goroutines made scanning fast, but debugging concurrent code requires care.
- Testing Saves the Day: Edge cases like unreachable hosts and large port ranges were caught early.
Viva la Programación
With this port scanner, you’ve mastered TCP handshakes and built a tool that’s both powerful and educational. Want to dig deeper? Fork this code, tweak it, and share your findings. And don’t forget: in programming, the revolution never ends.
¡Hasta la próxima! 🚀